By default, Codeigniter v3.1.13 already has a composer.json
file in root project, but it doesn’t have vendor
folder yet.
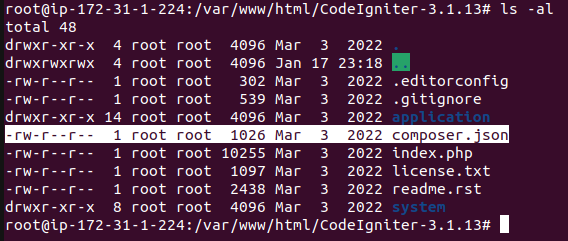
Now, if we want to install a library using composer, how?
- First, you have to install composer on your server
- Then running command
composer require
to install any library.
Install composer
For the information, i use Ubuntu 24.04 and PHP 8.0.3 here.
We are going to install composer.phar inside CodeIgniter-3.1.13 folder. So navigate to its folder.
cd /var/www/html/CodeIgniter-3.1.13
Install composer with these commands:
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
php -r "if (hash_file('sha384', 'composer-setup.php') === 'dac665fdc30fdd8ec78b38b9800061b4150413ff2e3b6f88543c636f7cd84f6db9189d43a81e5503cda447da73c7e5b6') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;"
php composer-setup.php
php -r "unlink('composer-setup.php');"

Now, you have composer.phar
file.
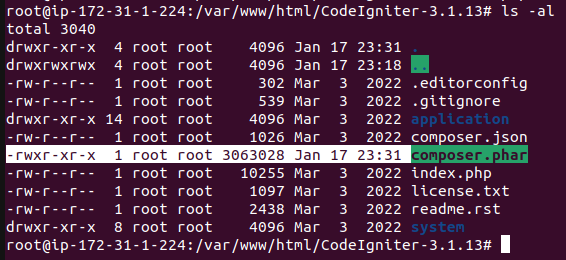
Install a library
Here, we are going to test installing a library. I use Guzzle PHP HTTP client.
php composer.phar require guzzlehttp/guzzle
If it returns some errors like this, you have to enable php module (php-dom)
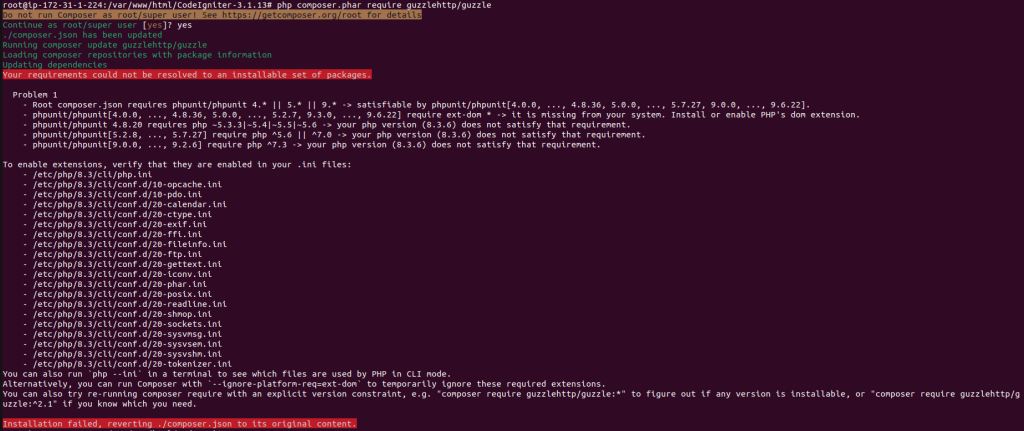
install php-dom:
apt-get install php-dom
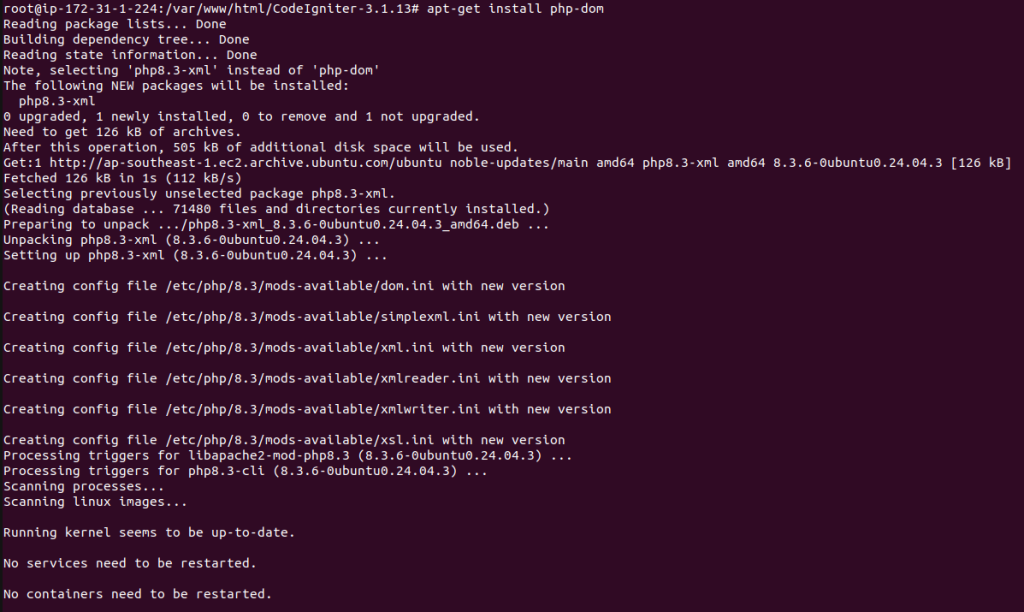
Now, let’s install guzzle again
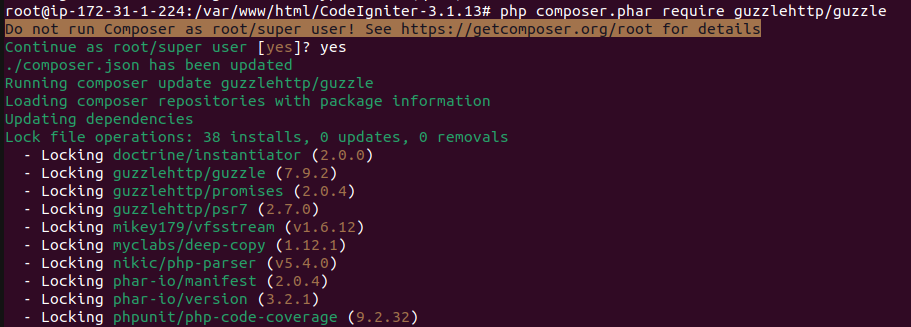
By this time, if you check root folder it will have vendor
folder, and guzzle stored in it.
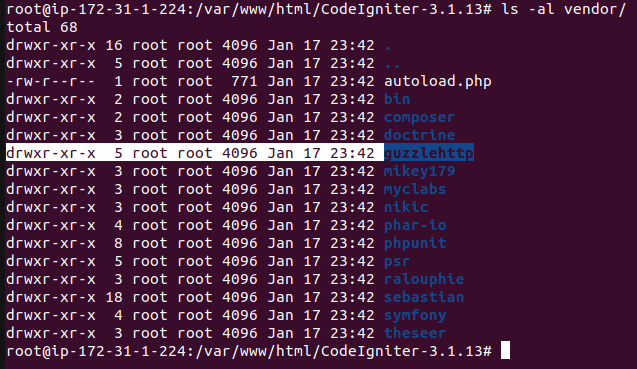
How to use installed library?
After guzzle installed, the next question is how to use that library?
To use the libary, first we need to load vendor/autoload.php
into CodeIgniter-3.1.13/application/config/config.php
Open config.php and modify this line:
$config['composer_autoload'] = FCPATH.'vendor/autoload.php';
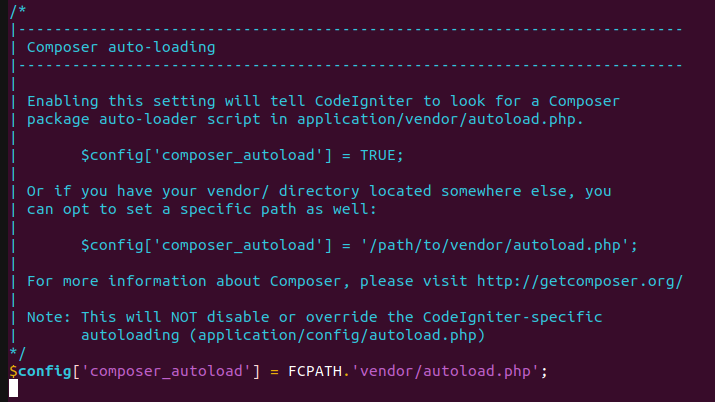
There are 2 ways to write guzzle codes into your controller:
- Using
use GuzzleHttp\Client;
outside Class - Using
new \GuzzleHttp\Client();
inside function block
Using use GuzzleHttp\Client;
outside Class
Open default controller (Welcome.php) and change the codes in it:
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
use GuzzleHttp\Client;
class Welcome extends CI_Controller {
public function index()
{
$client = new Client();
$response = $client->request('GET', 'https://api.github.com/repos/guzzle/guzzle');
echo $response->getStatusCode(); // 200
echo $response->getHeaderLine('content-type'); // 'application/json; charset=utf8'
echo $response->getBody(); // '{"id": 1420053, "name": "guzzle", ...}'
}
}
Run on your browser:
http://<your ip public>/Codeigniter-3.1.13/
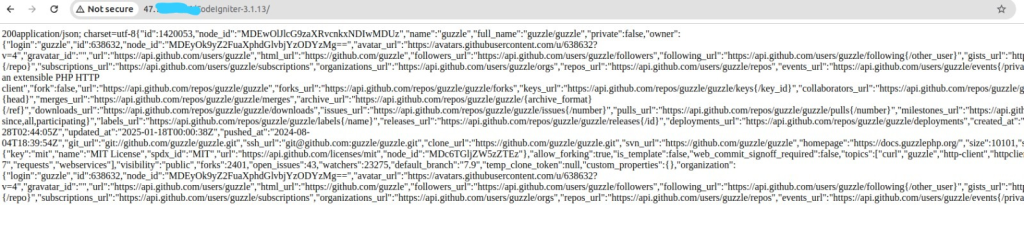
Using new \GuzzleHttp\Client();
inside function block
Instead of using use
outside the Class, you can load guzzle inside the function
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Welcome extends CI_Controller {
public function index()
{
$client = new \GuzzleHttp\Client();
$response = $client->request('GET', 'https://api.github.com/repos/guzzle/guzzle');
echo $response->getStatusCode(); // 200
echo $response->getHeaderLine('content-type'); // 'application/json; charset=utf8'
echo $response->getBody(); // '{"id": 1420053, "name": "guzzle", ...}'
}
}
The results:
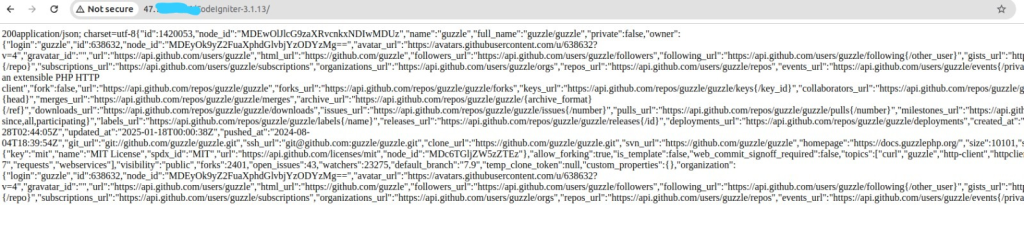
Conclusions
- We use Ubuntu 24.04 and PHP 8.0.3 in this tutorial
- To install 3rd party library using composer, we need to install
composer.phar
in Codeigniter 3.1.13 - To load vendor library into Codeigniter, configure it in
Codeigniter-3.1.13/application/config/config.php
in line$config['composer_autoload'] = FCPATH.'vendor/autoload.php';
- There are 2 ways to load guzzle in your controller:
- using
use GuzzleHttp\Client;
outside the Class - load directly in your function
new \GuzzleHttp\Client();
- using
- If there are some errors during installing a library using
php composer.phar require
, probably you need to install some php-extension (because some libraries depend on some php-extension)